React can be downloaded directly to use, download the package also provides a lot of examples of learning.
This tutorial uses React version 15.4.2, you can in the official website http://facebook.github.io/react/ download the latest version.
You can also use BootCDN's React CDN library directly, as follows:
< Script Src = " https://cdn.bootcss.com/react/15.4.2/react.min.js " > </ script >
< Script Src = " https://cdn.bootcss.com/react/15.4.2/react-dom.min.js " > </ script >
< Script Src = " https://cdn.bootcss.com/babel-standalone/6.22.1/babel.min.js " > </ script >
Use examples
The following example outputs Hello, world!
React instance
< ! DOCTYPE Html >
< Html >
< Head >
< Meta Charset = " UTF-8 " />
< Title > Hello React! </ Title >
< Script Src = " https://cdn.bootcss.com/react/15.4.2/react.min.js " > </ script >
< Script Src = " https://cdn.bootcss.com/react/15.4.2/react-dom.min.js " > </ script >
< Script Src = " https://cdn.bootcss.com/babel-standalone/6.22.1/babel.min.js " > </ script >
</ Head >
< Body >
< Div Id = " example " > </ div >
< Script Type = " text / babel " > ReactDOM.render ( < h1 > Hello, world! </ H1 > , document.getElementById ('example')); </ script >
</ Body >
</ Html >
try it"
Example Analysis:
In the example we have introduced three libraries: react.min.js, react-dom.min.js and babel.min.js:
- React.min.js - React's core library
- React-dom.min.js - Provides DOM-related functionality
- Babel.min.js - Babel can convert the ES6 code to the ES5 code so that we can execute the React code on the ES6 browser that does not currently support it. Babel has embedded support for JSX. By using Babel and the babel-sublime package, you can let the grammar rendering of the source code rise to a whole new level.
ReactDOM . Render ( < h1 > Hello , world ! </ H1 >, document . GetElementById ( ' example ' )
) ;
The above code inserts an h1 header into the id = "example" node.
note:If we need to use JSX, the <type> tag of the <script> tag needs to be set to text / babel.
React is used by npm
If your system does not support Node.js and NPM you can refer to our Node.js tutorial .
We recommend using the CommonJS module system in React, such as browserify or webpack, this tutorial uses webpack.
Domestic use npm speed is very slow, you can use Taobao custom cnpm (gzip compression support) command line tool instead of the default npm:
$ Npm install -g cnpm --registry = https: //registry.npm.taobao.org $ Npm config set registry https://registry.npm.taobao.org
So you can use the cnpm command to install the module:
$ Cnpm install [name]
More information can be found at: http://npm.taobao.org/ .
Use the create-react-app to quickly build the React development environment
Create-react-app is from Facebook, through which we can quickly build React development environment without configuration.
Create-react-app is automatically created based on Webpack + ES6.
Execute the following command to create the project:
$ Cnpm install -g create-react-app $ Create-react-app my-app $ Cd my-app / $ Npm start
In the browser to open http: // localhost: 3000 / , the results as shown below:
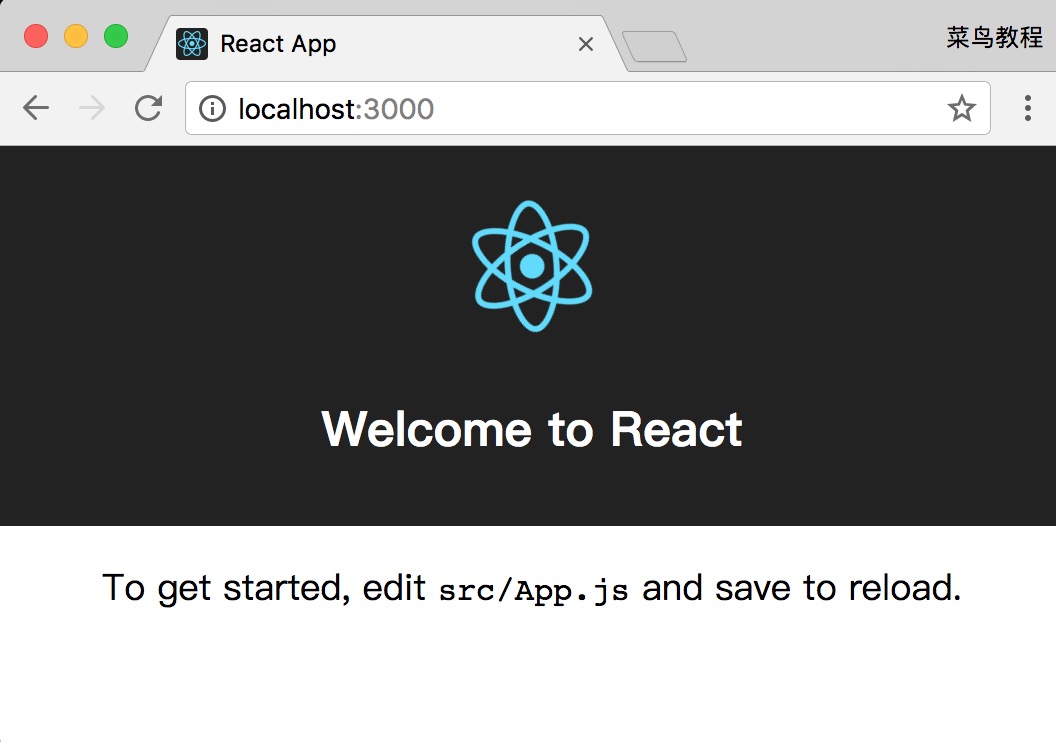
The directory structure of the project is as follows:
My-app / README.md Node_modules / Package.json .gitignore Public / Favicon.ico Index.html Src / App.css App.js App.test.js Index.css Index.js Logo.svg
Try to modify src / app.js file code:
Src / app.js
Import React , { Component } From ' React ' ; import Logo From ' ./logo.svg ' ; import ' ./App.css ' ; class App Extends Component {
Render ( ) {
Return ( < Div ClassName = " App " > < div ClassName = " App-header " > < img Src = { logo } ClassName = " App-logo " Alt = " logo " /> < h2 > Welcome to the rookie tutorial </ h2 > </ div > < p ClassName = " App-intro " > You can modify it in the < code > src / app . Js </ code > file. </ P > </ div > ) ; }
}
Export Default App ;
Modify, open http: // localhost: 3000 / (usually automatically refresh), the output is as follows:
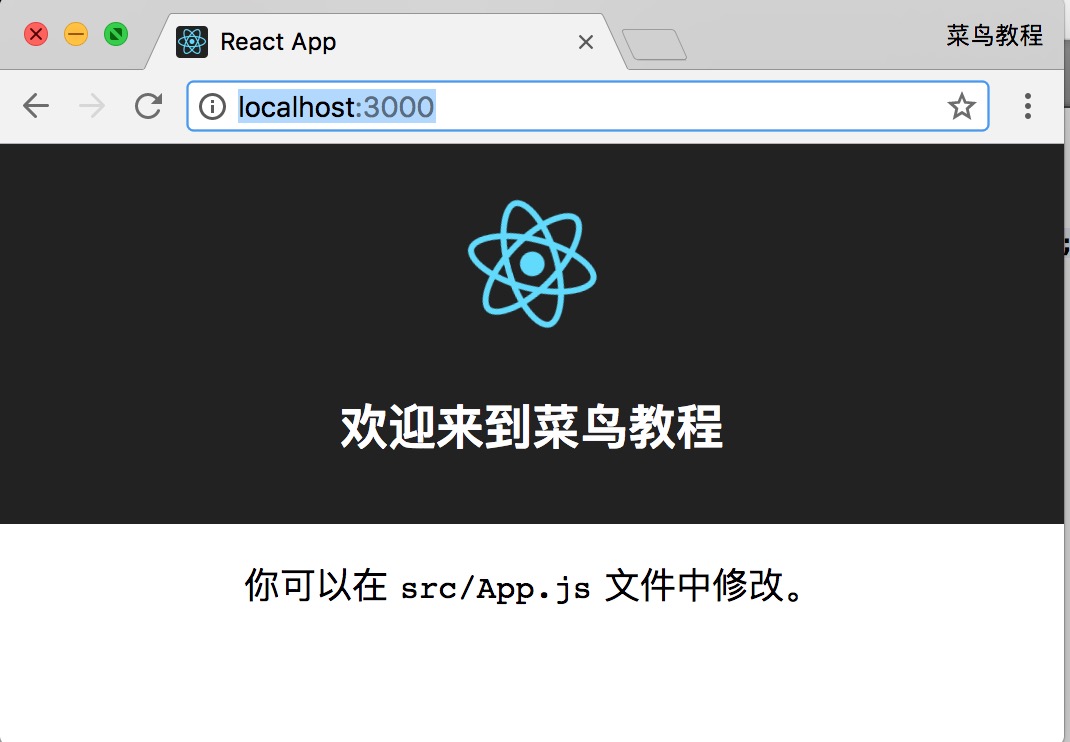
EmoticonEmoticon